How to Optimize Your Code for Better Performance: A Developer's Guide
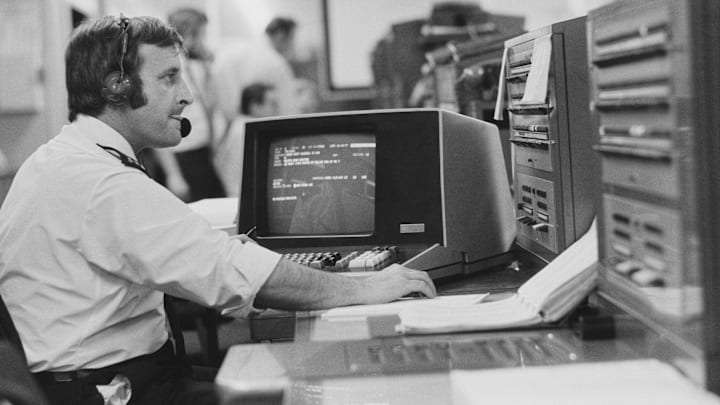
Writing functional code is just the beginning. As applications scale, performance becomes a critical factor, impacting user experience, resource usage, and overall system efficiency. Optimizing your code ensures that it runs faster, consumes fewer resources, and adapts smoothly to increasing demands. Whether you’re building a web app, mobile application, or software for embedded systems, these principles and strategies will help you write efficient and performant code.
Understand the Problem Before Optimizing
Before diving into optimization, identify the specific issues affecting performance. Is your application slow because of excessive memory usage? Are certain operations taking too long to execute? Use profiling tools to pinpoint bottlenecks and prioritize improvements where they matter most. Premature optimization—tweaking parts of your code without clear evidence of a problem—can waste time and complicate your codebase unnecessarily. Always start with a clear understanding of what needs to be optimized.
Strategies for Code Optimization
Choose the Right Algorithm
The choice of algorithm can dramatically affect performance. An inefficient algorithm, even with optimal implementation, will underperform compared to a well-chosen one. Use sorting, searching, or data manipulation algorithms with the best complexity for your use case. Prefer algorithms with lower computational complexity when handling large datasets.
Optimize Data Structures
Selecting the right data structure is just as important as choosing the right algorithm. Use structures that match your requirements. Hash maps or dictionaries are ideal for fast lookups, arrays for sequential data access, and linked lists for dynamic memory allocation when insertion and deletion are frequent. Efficient data structures reduce overhead and improve the speed of operations like insertion, deletion, and traversal.
Reduce Redundant Calculations
Avoid performing the same calculations multiple times. Cache results of expensive operations that are reused frequently. Use memoization to store results of function calls for reuse, and apply dynamic programming techniques where applicable to eliminate repeated computations.
Minimize I/O Operations
Input and output operations, including file handling and database queries, are often slow. Optimize them by bundling multiple I/O requests into fewer operations, reading and writing data in bulk rather than line by line, and using asynchronous or non-blocking I/O to handle tasks in parallel.
Use Efficient Loops
Loops are common culprits in performance issues. Optimize them by reducing unnecessary iterations, avoiding complex operations within the loop body, and using language-specific constructs that are often more efficient. For instance, list comprehensions can be faster than traditional loops in some languages.
Manage Memory Wisely
Efficient memory usage prevents unnecessary allocation and deallocation overhead. Reuse objects instead of creating new ones wherever possible, use appropriate data types, and avoid using large or complex structures for simple values. In languages without automatic garbage collection, explicitly free up memory to prevent leaks.
Parallelize Tasks
Take advantage of multi-core processors by parallelizing tasks. Split large jobs into smaller ones that can run concurrently. Use tools and frameworks that support parallel computing, like threading or multiprocessing libraries.
Optimize Database Queries
Database interactions often slow down applications. Optimize your queries by indexing columns that are frequently queried, avoiding SELECT * by specifying only the needed columns, and using prepared statements and batch processing for repeated operations.
Profile and Benchmark Your Code
Use profiling tools to measure the execution time of functions and identify bottlenecks. Benchmark different approaches to find the most efficient solution for critical parts of your code. Regular profiling helps you track performance regressions as your codebase evolves.
Language-Specific Optimizations
While the general principles of optimization apply across all programming languages, specific languages offer unique tools and techniques. For example, in Python, built-in libraries like NumPy are optimized for speed, while in JavaScript, minimizing DOM manipulations and using debouncing or throttling for event handlers can significantly improve performance. In C++, leveraging inline functions and preferring stack allocation over heap allocation can enhance efficiency. Understanding the nuances of your language allows you to exploit its strengths while avoiding common pitfalls.
Best Practices for Sustainable Optimization
Write Clean and Readable Code First
Always prioritize code readability over premature optimization. Start with simple, clear code and optimize only when necessary. Maintainability is just as important as performance.
Keep It Modular
Break your application into smaller, independent modules. This not only makes debugging easier but also allows you to optimize specific parts without affecting the entire system.
Leverage Built-In Libraries
Standard libraries and frameworks are often optimized for performance and reliability. Whenever possible, use these tools instead of writing custom implementations.
Test Across Real-World Scenarios
Optimize based on realistic workloads, not just idealized test cases. Simulate real-world usage patterns to ensure your improvements are effective under actual conditions.
When to Stop Optimizing
Optimization can be a never-ending process. However, there’s a point where the effort outweighs the benefit. Once your application meets acceptable performance levels for your users and use case, further optimization may not be worth the trade-offs in complexity and development time.
Conclusion
Optimizing your code is about finding the balance between speed, efficiency, and maintainability. By focusing on algorithms, data structures, and thoughtful memory management, you can significantly improve performance. Pair these techniques with regular profiling and a deep understanding of your programming environment to build software that not only works but excels. Remember, good performance isn’t just about writing faster code—it’s about delivering better experiences.