How to Implement Security Best Practices in Your Code
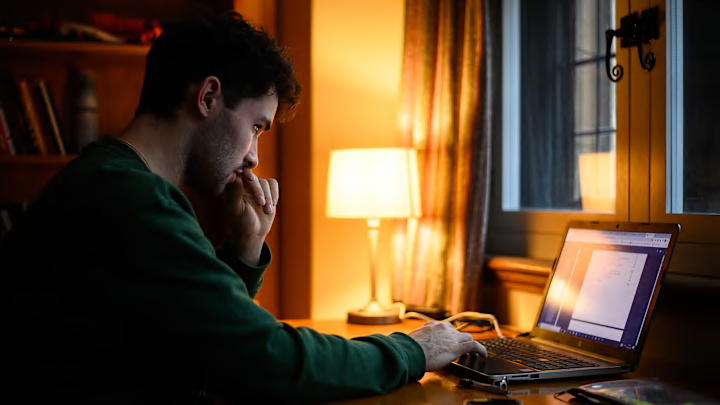
In the ever-evolving digital landscape, security breaches can have catastrophic consequences for businesses and individuals alike. Writing secure code is not just a best practice—it’s a necessity. As developers, integrating security measures into the development process is essential to safeguard applications and protect user data. Here’s how to implement security best practices in your code to minimize vulnerabilities and build robust, secure applications.
1. Validate Input
Always validate and sanitize input from users to prevent malicious data from entering your application. Input validation ensures that data conforms to expected formats, such as email addresses or numerical values. Sanitization removes or escapes dangerous characters, reducing the risk of injection attacks.
For example, in web applications, failure to validate input could lead to SQL injection, where attackers manipulate database queries through user input. Use parameterized queries or prepared statements to mitigate this risk.
2. Use Secure Authentication and Authorization
Implement robust authentication mechanisms to verify user identities. Avoid building custom authentication systems unless absolutely necessary—use proven libraries and frameworks instead.
Best practices for authentication include enforcing strong password policies, hashing passwords using algorithms like bcrypt, and implementing multi-factor authentication (MFA).
Authorization ensures that users only access resources they’re permitted to use. Use the principle of least privilege to minimize access levels, and regularly audit permissions to avoid accidental exposure.
3. Encrypt Sensitive Data
Protect sensitive data by encrypting it both in transit and at rest. Use HTTPS (TLS) to encrypt data transmitted over networks, preventing interception by attackers. For stored data, use strong encryption algorithms such as AES-256.
Never store sensitive information, such as passwords, in plaintext. Always hash passwords before saving them to a database, and add a unique salt to each hash to enhance security.
4. Avoid Hardcoding Secrets
Never hardcode sensitive information like API keys, database credentials, or encryption keys directly into your codebase. This exposes them to unauthorized access if your code is leaked or compromised.
Instead, store secrets securely using environment variables or secret management tools like AWS Secrets Manager, Azure Key Vault, or HashiCorp Vault.
5. Regularly Update Dependencies
Using third-party libraries and frameworks can speed up development, but outdated or unpatched dependencies often contain vulnerabilities. Regularly audit your dependencies and update them to their latest stable versions.
Tools like Dependabot, npm audit, or OWASP Dependency-Check can help identify and mitigate risks associated with vulnerable packages.
6. Implement Error Handling Carefully
Proper error handling is crucial for both usability and security. Avoid exposing detailed error messages to end-users, as these can reveal sensitive information about your application’s structure.
Log detailed errors in a secure location for developers to review, but show generic error messages to users. For example, avoid displaying database errors directly to users, which could provide clues for exploitation.
7. Protect Against Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) occurs when attackers inject malicious scripts into a web application. To prevent XSS:
- Escape user input before rendering it in the browser.
- Use content security policies (CSP) to restrict allowed sources of scripts.
- Rely on frameworks that include built-in XSS protections.
Sanitizing inputs and using secure methods for rendering data can significantly reduce the risk of XSS attacks.
8. Secure API Endpoints
APIs are often a target for attackers because they provide direct access to application functionality and data. Secure your API endpoints by:
- Using authentication tokens, such as OAuth2 or JSON Web Tokens (JWT).
- Validating and sanitizing all API requests.
- Implementing rate limiting to prevent abuse, such as brute-force attacks.
Ensure that sensitive operations are protected by HTTPS, and avoid exposing unnecessary API endpoints.
9. Monitor and Log Activity
Implement logging to track user activity and application behavior. Securely store logs to detect suspicious behavior, such as repeated failed login attempts or unexpected API calls.
Use monitoring tools to gain real-time insights into your application’s security status. Proactive monitoring allows you to respond quickly to potential breaches or vulnerabilities.
10. Conduct Regular Security Testing
Regularly test your code and application for vulnerabilities using manual reviews and automated tools. Common methods include:
- Static Application Security Testing (SAST): Analyzes source code for security flaws.
- Dynamic Application Security Testing (DAST): Tests a running application for vulnerabilities.
- Penetration Testing: Simulates real-world attacks to identify weaknesses.
These tests help uncover issues like insecure data handling, weak encryption, and misconfigurations.
11. Educate Your Team
Security is a team effort. Educate all developers, testers, and stakeholders about secure coding practices. Stay updated on the latest security trends, vulnerabilities, and mitigation techniques through resources like OWASP and industry blogs.
Encourage a culture of security by integrating it into every stage of the development lifecycle, from design to deployment.
12. Follow Security Standards
Adhere to recognized security standards and guidelines, such as those provided by OWASP, NIST, and ISO/IEC. These frameworks offer best practices and checklists to ensure your application meets industry security benchmarks.
Conclusion
Secure coding is essential for protecting your application, users, and reputation. By following these best practices, you can minimize vulnerabilities, reduce the risk of breaches, and ensure a robust and secure codebase. Security should never be an afterthought—make it an integral part of your development process and continuously improve to stay ahead of evolving threats.