How to Create RESTful APIs in Node.js
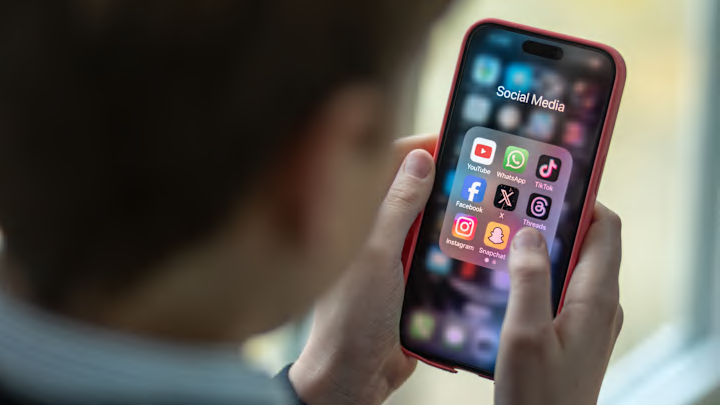
In today’s digital landscape, APIs (Application Programming Interfaces) are the backbone of modern web and mobile applications, enabling seamless communication between different systems. RESTful APIs, in particular, have become the standard for building scalable and flexible web services. Using Node.js, you can create robust RESTful APIs efficiently, thanks to its lightweight runtime and wide array of libraries. This guide walks you through the process of building a RESTful API in Node.js.
What is a RESTful API?
A RESTful API (Representational State Transfer) is a design pattern for web services that uses HTTP methods to interact with resources. These methods typically include:
- GET: Retrieve data from the server.
- POST: Create new data on the server.
- PUT: Update existing data.
- DELETE: Remove data from the server.
RESTful APIs follow principles like statelessness, resource-based URL structures, and standard HTTP status codes for communication.
Why Use Node.js for RESTful APIs?
Node.js is an excellent choice for building RESTful APIs due to its:
- Lightweight Nature: Handles multiple requests efficiently with its non-blocking, event-driven architecture.
- Rich Ecosystem: A vast library of modules, like Express, makes API development faster and easier.
- Single Language: Use JavaScript on both the client and server sides, simplifying development.
Steps to Create a RESTful API in Node.js
1. Set Up Your Environment
Before starting, ensure you have Node.js and npm (Node Package Manager) installed on your machine. Create a new directory for your project and initialize it with:
bash
Copy code
npm init -y
This generates a package.json file to manage your dependencies.
2. Install Required Packages
The most popular framework for building APIs in Node.js is Express. Install it using:
bash
Copy code
npm install express
You might also need additional packages for specific functionalities, such as:
- Body Parsing: body-parser to handle JSON requests (though Express has built-in support for JSON parsing in modern versions).
- Database Integration: Packages like mongoose for MongoDB or pg for PostgreSQL.
3. Create the Basic Server
Start by creating a basic Express server that listens for requests.
4. Define API Routes
RESTful APIs revolve around resources. For example, if you’re building a "Users" API, you’ll define routes to handle user-related operations:
- GET /users: Fetch all users.
- GET /users/:id: Fetch a single user by ID.
- POST /users: Create a new user.
- PUT /users/:id: Update a user.
- DELETE /users/:id: Delete a user.
Each route corresponds to a function that handles the logic for that operation.
5. Handle Requests and Responses
Use Express’s route handlers to manage requests. For example, when a client sends a GET request to /users, the server retrieves a list of users and sends it back as a JSON response.
6. Connect to a Database
Most RESTful APIs interact with a database to store and retrieve data. Common databases include:
- MongoDB: A NoSQL database that works well with Node.js, often accessed using the mongoose library.
- PostgreSQL/MySQL: Relational databases accessed using libraries like pg or sequelize.
Set up database models to define the structure of your data, then use CRUD operations (Create, Read, Update, Delete) to manipulate it.
7. Implement Middleware
Middleware functions in Express process requests and responses. Examples include:
- Logging request details using libraries like morgan.
- Parsing incoming JSON data with built-in middleware.
- Handling authentication and authorization.
8. Add Error Handling
Implement error handling to return meaningful responses when something goes wrong. Use standardized HTTP status codes like:
- 200 OK: Request was successful.
- 201 Created: Resource was successfully created.
- 400 Bad Request: The request had invalid data.
- 404 Not Found: The requested resource doesn’t exist.
- 500 Internal Server Error: Something went wrong on the server.
Catch errors in your routes or database queries and send appropriate responses to the client.
9. Test Your API
Test your API using tools like Postman or cURL. These tools allow you to send HTTP requests to your API and verify the responses. Write automated tests using libraries like Jest or Mocha to ensure your API works as expected.
10. Deploy Your API
When your API is ready, deploy it to a hosting platform like AWS, Heroku, or Vercel. Use process managers like PM2 to keep your API running smoothly in production.
Best Practices for RESTful APIs
- Follow REST Principles: Use clear and consistent naming conventions for routes, like /resources or /resources/:id.
- Validate Input: Use libraries like Joi or express-validator to ensure incoming data is correct.
- Secure Your API: Implement authentication and authorization using tokens (e.g., JWT) or OAuth2.
- Paginate Results: For large datasets, provide paginated responses to improve performance.
- Document Your API: Use tools like Swagger or Postman to create interactive API documentation for developers.
Conclusion
Creating a RESTful API in Node.js is an efficient way to build scalable and flexible web services. With its non-blocking architecture and rich ecosystem of libraries, Node.js simplifies the process of handling HTTP requests, interacting with databases, and managing middleware. By following best practices and continually improving your API, you can ensure a robust and secure service that meets the needs of your users.