From Zero to Hero in JavaScript: Mastering the Web’s Language of Power
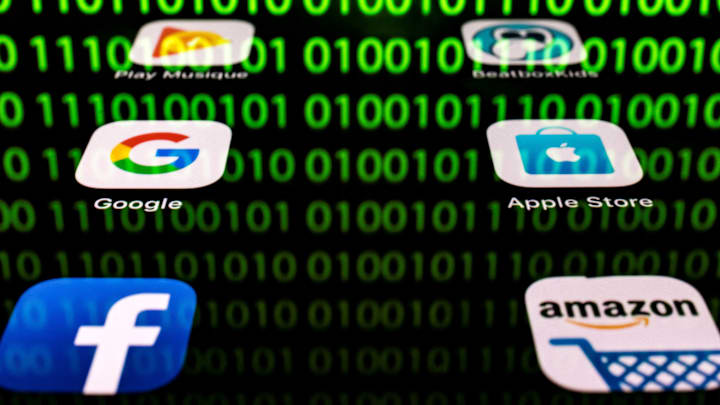
JavaScript—often abbreviated as JS—is the heartbeat of the web. It’s the language that brings websites to life, enabling dynamic content, interactivity, and the smooth experiences we’ve come to expect. Whether you’re just starting your coding journey or looking to expand your programming repertoire, mastering JavaScript can transform you from a novice coder into a full-fledged web development hero. Here’s how you can conquer JavaScript, step by step.
The JavaScript Origin Story: Why It’s Essential
Back in 1995, JavaScript was created in just ten days by Brendan Eich to add interactivity to static web pages. Since then, it has evolved from a humble scripting language into a powerful ecosystem for front-end, back-end, and even mobile app development.
Today, JavaScript is everywhere—used by nearly 98% of websites and powered by frameworks like React, Angular, and Vue. Its versatility and ubiquity make it one of the most valuable skills for any aspiring developer.
Step 1: Understand the Basics
To embark on your hero’s journey, you need a solid foundation. Start by familiarizing yourself with JavaScript’s syntax, rules, and core concepts.
Variables and Data Types
JavaScript is a loosely typed language, allowing you to declare variables using var, let, or const. Explore its various data types:
- Primitives like strings, numbers, and booleans.
- Special types like null and undefined.
- Objects, arrays, and functions.
Control Structures
Learn how to control the flow of your program using conditionals (if, else, switch) and loops (for, while, forEach). These are the building blocks of logic in your code.
Functions: The Real Heroes
Functions are the lifeblood of JavaScript. Master how to declare functions, pass arguments, and return values. Get comfortable with ES6+ arrow functions for cleaner, modern syntax.
Step 2: Explore the DOM—The Web’s Playground
The Document Object Model (DOM) is your bridge to interactivity. It represents the structure of a web page, allowing JavaScript to manipulate elements dynamically.
- Selecting Elements: Use getElementById, querySelector, or getElementsByClassName to grab elements.
- Changing Content: Modify text, attributes, or styles with properties like innerHTML and style.
- Event Listeners: Make pages interactive by responding to user actions like clicks, hovers, or keyboard inputs using addEventListener.
Step 3: Level Up with ES6+ Features
JavaScript’s modern updates have introduced powerful features to streamline your code.
- Template Literals: Use backticks () for multi-line strings and embedding variables with ${}`.
- Destructuring: Simplify object and array manipulation.
- Spread and Rest Operators: Copy or merge objects and arrays effortlessly.
- Promises and Async/Await: Manage asynchronous operations like API calls with clean, readable syntax.
Step 4: Dive into Debugging
Bugs are inevitable, but a hero knows how to defeat them. Learn to debug JavaScript effectively with tools like:
- console.log(): Your trusty companion for tracking values and states.
- Browser Developer Tools: Chrome and Firefox offer robust debugging features, from breakpoints to performance insights.
Step 5: Enter the Framework Zone
Once you’re comfortable with vanilla JavaScript, it’s time to explore libraries and frameworks that supercharge your development.
- Front-End Frameworks: React, Angular, and Vue streamline UI development with reusable components and state management.
- Back-End with Node.js: Take JavaScript to the server side to build APIs, handle databases, and create full-stack applications.
- Mobile Development: Use React Native or Ionic to craft cross-platform mobile apps using JavaScript.
Step 6: Learn by Building
Theory is essential, but practice makes the hero. Build projects to solidify your knowledge:
- Beginner: Create a to-do list or a simple calculator.
- Intermediate: Develop a weather app using an API like OpenWeather.
- Advanced: Build a full-stack application with user authentication and a database.
Step 7: Keep Up with the Ecosystem
JavaScript evolves rapidly, so staying updated is critical. Follow resources like:
- MDN Web Docs: The go-to reference for all things JavaScript.
- YouTube Channels and Blogs: Tutorials from creators like Traversy Media or Dev Ed.
- Communities: Join forums, Slack groups, or GitHub repositories to connect with fellow learners and developers.
Step 8: Adopt Best Practices
Every hero needs discipline. Follow best practices to write clean, maintainable code:
- Use consistent formatting and indentation.
- Avoid global variables to reduce conflicts.
- Comment code sparingly but effectively to enhance readability.
- Write modular code by breaking large scripts into smaller, reusable functions or modules.
From Novice to JavaScript Hero
JavaScript mastery isn’t an overnight achievement—it’s a journey of persistence, curiosity, and creativity. As you learn, experiment, and solve problems, you’ll unlock the full potential of the web’s most powerful language.
Ready to don your hero’s cape? The world of JavaScript awaits. Remember, every bug squashed, every feature implemented, and every app launched is another step toward greatness. Now, go forth and code!