Data Structures Demystified A Crash Course
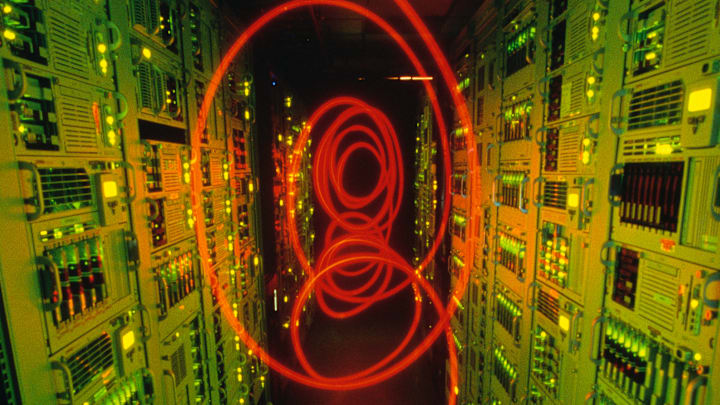
In the intricate tapestry of modern programming, data structures are the threads that hold everything together. Whether it’s organizing massive datasets or optimizing search algorithms, these fundamental tools are the unsung heroes of software development. But fear not—while the term might conjure images of dense textbooks and cryptic code, this crash course will demystify data structures and unveil their elegant simplicity.
What Are Data Structures and Why Should You Care?
At their core, data structures are methods of organizing and storing data to enable efficient access and modification. They’re the toolkits programmers use to solve problems, whether it’s matching ride-share drivers with passengers or predicting the next trending Netflix series. Without them, our digital world would resemble a cluttered attic, where every search involves rummaging through piles of irrelevant junk.
But their true magic lies in their versatility. The choice of the right data structure can transform a sluggish algorithm into a high-speed marvel. Think of it like choosing the right vessel for a journey: a canoe for a calm river, a speedboat for a race, and a submarine for the depths.
Arrays and Linked Lists: The Basics
Arrays are the bread and butter of data structures—simple, reliable, and easy to grasp. They store elements in a contiguous block of memory, like books neatly arranged on a shelf. The perk? Instant access to any book, provided you know its position.
Linked lists, on the other hand, are like treasure maps. Each element, or node, contains the data and a pointer to the next node. While they lack the instant accessibility of arrays, they shine when it comes to flexibility. Adding or removing elements is a breeze—no need to reshuffle an entire bookshelf.
Stacks and Queues: Keeping Things in Order
Next up, we have stacks and queues, which deal with data in a sequential manner. Stacks operate on the principle of Last In, First Out (LIFO), much like a stack of plates at a buffet. Only the top plate is accessible, making stacks perfect for tasks like undo operations or navigating through a browser’s history.
Queues, by contrast, follow First In, First Out (FIFO)—think of a line at a coffee shop. Whoever arrives first gets served first. They’re ideal for scenarios where order matters, such as managing tasks in a printer queue or scheduling processes in an operating system.
Trees and Graphs: Climbing the Ladder of Complexity
When data relationships grow intricate, arrays and lists often fall short. Enter trees and graphs—the data structures that branch out (literally) to handle hierarchical and networked information.
Trees, with their roots, branches, and leaves, are ideal for representing hierarchical data, like organizational charts or file systems. Binary search trees take it up a notch, enabling rapid searches by maintaining sorted data.
Graphs, meanwhile, connect data points, or vertices, with edges, forming webs of relationships. Whether mapping social networks, modeling city traffic, or optimizing delivery routes, graphs provide the structure for some of the most complex problems in computer science.
Hash Tables: The Ultimate Lookup Tool
Hash tables are the Swiss Army knives of data structures, providing lightning-fast lookups. By using a hash function to map keys to indices in an array, they allow near-instant access to data. They’re the secret behind the blazing speed of search engines and database indexing.
However, like all superheroes, they have their Achilles’ heel: collisions. When two keys map to the same index, creative solutions like chaining or open addressing come to the rescue.
Choosing the Right Data Structure: Art Meets Science
Selecting the perfect data structure isn’t a one-size-fits-all scenario. It’s a blend of understanding the problem, analyzing trade-offs, and sometimes making compromises. For instance, arrays are great for fixed-size collections, but dynamic datasets might call for linked lists. Similarly, trees excel in searching and sorting, while graphs are indispensable for mapping relationships.
Conclusion: The Beauty of Structure
Data structures may seem daunting at first glance, but they’re the unsung architects of the digital age. Each type has its strengths and weaknesses, and mastering them is akin to learning the tools of a craftsperson. Whether you’re a budding coder or an experienced programmer, understanding data structures equips you with the power to write smarter, faster, and more efficient code.
So, the next time you hear terms like "queue" or "hash table," don’t panic. Instead, marvel at their elegance and remember: in the world of programming, structure isn’t just a tool—it’s the key to unlocking endless possibilities.