Breaking Down Algorithms: A Beginner’s Guide
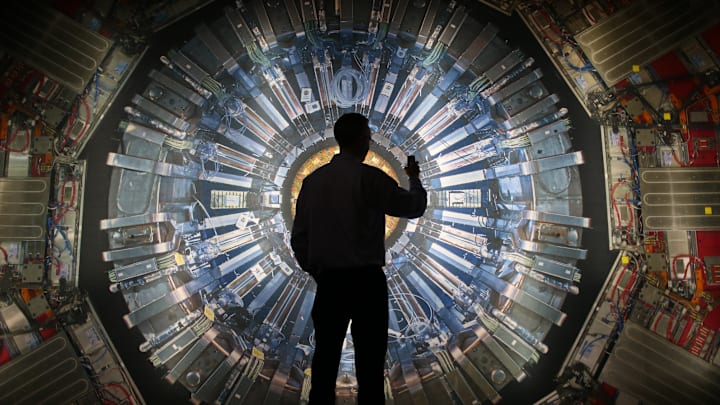
Algorithms are the foundation of computer science, powering everything from search engines to recommendation systems. For beginners, the term "algorithm" can feel intimidating, conjuring up images of complex math and indecipherable code. However, at its core, an algorithm is simply a set of instructions to solve a problem. This guide breaks down the basics of algorithms, explains why they matter, and introduces you to some key concepts to get you started.
What is an Algorithm?
An algorithm is a step-by-step procedure for solving a specific problem or performing a task. It’s like a recipe in cooking: a list of instructions that, when followed, produce the desired result. Algorithms can be written in plain language, pseudocode, or implemented in programming languages.
For example, an algorithm for making a cup of tea might look like this:
- Boil water.
- Place a tea bag in a cup.
- Pour boiling water into the cup.
- Let the tea steep for 3-5 minutes.
- Remove the tea bag and add sugar or milk if desired.
In computing, algorithms are designed to solve problems like sorting a list, finding the shortest path in a graph, or compressing data.
Why Are Algorithms Important?
Algorithms are crucial because they determine how efficiently a task is performed. Different algorithms can solve the same problem, but some are faster, use less memory, or are easier to implement.
For example, consider sorting a list of numbers. While there are many sorting algorithms (e.g., bubble sort, quicksort, merge sort), each has its strengths and weaknesses depending on the size of the list and the context in which it’s used. Choosing the right algorithm can mean the difference between waiting seconds or hours for your program to finish.
Key Concepts in Algorithms
1. Input and Output
Every algorithm starts with input (data to work with) and produces output (the solution). For example, in a search algorithm, the input might be a list of names and a target name to find, and the output would be the position of the target name in the list.
2. Efficiency
Efficiency measures how well an algorithm performs, typically in terms of time (how fast it runs) and space (how much memory it uses). Algorithms are analyzed using Big-O notation, which describes their performance in terms of input size. Common Big-O complexities include:
- O(1): Constant time, regardless of input size.
- O(n): Linear time, grows proportionally with input size.
- O(n²): Quadratic time, grows quickly as input size increases.
3. Steps and Logic
Algorithms must be clear and unambiguous, with steps that can be executed in sequence. Each step should lead logically to the next until the problem is solved.
4. Iteration and Recursion
Many algorithms use loops (iteration) or self-calling functions (recursion) to process data repeatedly until a condition is met. For example, a recursive algorithm might solve smaller sub-problems and combine their solutions to solve the larger problem.
Types of Algorithms
1. Sorting Algorithms
These arrange data in a specific order, such as ascending or descending. Common sorting algorithms include:
- Bubble Sort: Compares adjacent elements and swaps them if needed.
- Merge Sort: Divides the list into smaller parts, sorts them, and merges them back together.
- Quick Sort: Selects a pivot and partitions the list into smaller and larger elements before sorting.
2. Search Algorithms
These find specific data within a dataset. Examples include:
- Linear Search: Checks each element one by one until the target is found.
- Binary Search: Efficiently searches sorted lists by dividing the dataset in half with each step.
3. Graph Algorithms
These solve problems related to networks, such as finding the shortest path or detecting cycles. Examples include:
- Dijkstra’s Algorithm: Finds the shortest path between nodes in a weighted graph.
- Breadth-First Search (BFS): Explores nodes level by level.
- Depth-First Search (DFS): Explores as far down a branch as possible before backtracking.
4. Divide and Conquer
These algorithms break a problem into smaller sub-problems, solve them, and combine their solutions. Examples include merge sort and quicksort.
5. Dynamic Programming
This approach solves problems by breaking them into overlapping sub-problems and storing their solutions to avoid redundant work. Examples include the Fibonacci sequence and the knapsack problem.
Steps to Learn Algorithms as a Beginner
1. Understand the Problem
Start by thoroughly understanding the problem you want to solve. Break it down into smaller parts and think logically about how to address each piece.
2. Learn Pseudocode
Before jumping into code, practice writing algorithms in plain language or pseudocode. This helps you focus on the logic without worrying about syntax.
3. Implement Basic Algorithms
Start with simple algorithms like linear search or bubble sort. Write them in your preferred programming language to understand how they work in practice.
4. Study Algorithm Analysis
Learn how to evaluate the efficiency of algorithms using Big-O notation. This helps you compare and choose the best algorithm for a given task.
5. Explore Common Algorithms
Familiarize yourself with widely-used algorithms, such as sorting, searching, and graph traversal. Practice implementing them and solving related problems.
6. Solve Problems Regularly
Use platforms like LeetCode, HackerRank, or Codewars to practice algorithmic problem-solving. Gradually tackle more complex challenges to build your skills.
Tips for Success
- Focus on understanding the logic behind algorithms rather than memorizing code.
- Break complex algorithms into smaller parts and understand each step.
- Visualize algorithms using diagrams or step-by-step walkthroughs to see how data changes.
- Collaborate with others or seek help from online communities to improve your understanding.
Conclusion
Algorithms are the backbone of problem-solving in computer science and programming. By understanding their purpose, learning their basic principles, and practicing regularly, you can build a strong foundation that will serve you in countless coding scenarios. While the journey may seem challenging at first, breaking down algorithms into manageable concepts makes it an exciting and rewarding endeavor. Start small, stay curious, and let your algorithmic thinking grow.