Best Practices for Writing Clean Code: Crafting Clarity and Elegance
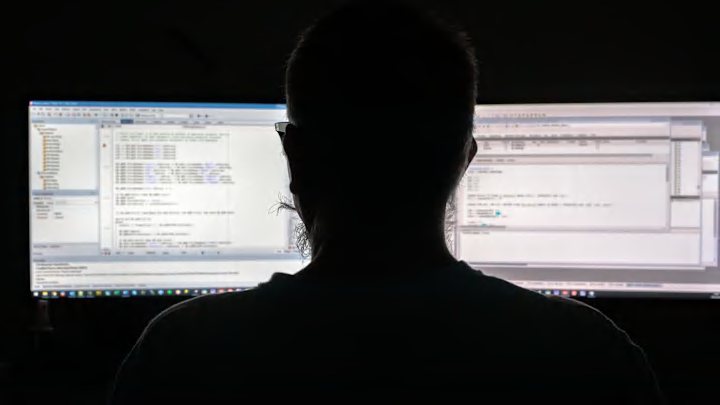
Clean code is more than just functional—it’s about creating code that’s easy to read, maintain, and build upon. Writing clean code isn’t just for others; it’s a favor to your future self, ensuring that your work remains accessible and adaptable. While mastery comes with experience, certain principles can help you develop professional-grade, maintainable code from the start.
Understand the Problem Before Writing Code
Before you type your first line, take the time to fully understand the requirements and goals of the task. Rushing into coding can lead to overly complex or poorly structured solutions. Think through the problem, plan the architecture, and consider edge cases. A well-thought-out plan often results in cleaner, more intuitive code.
Use Descriptive Names
The names you assign to variables, functions, and classes should clearly reflect their purpose. Avoid ambiguous or overly brief names. Instead, use terms that convey their role in the code. This practice ensures that others (and you) can quickly understand what each part of the program does, making your code self-explanatory.
Keep Functions and Methods Focused
Each function or method should perform a single, specific task. Overloading functions with multiple responsibilities makes them harder to understand, debug, and test. By breaking tasks into smaller, focused functions, you improve readability and reusability, which is the cornerstone of clean code.
Follow Consistent Naming Conventions
Adopt a consistent naming style and stick to it throughout your codebase. Whether it’s using camelCase, snake_case, or PascalCase, uniformity makes the code predictable and easier to read. Adhering to naming conventions improves collaboration, as team members can quickly align with the established structure.
Avoid Hardcoding Values
Hardcoding values directly into your code makes it inflexible and harder to update. Instead, define constants or use configuration files to store such values. This practice not only simplifies maintenance but also makes your code more adaptable to different environments or requirements.
Comment with Purpose
While clean code should be self-explanatory whenever possible, comments are still important for explaining the “why” behind complex logic. Avoid redundant or outdated comments, as they can confuse readers. Use comments sparingly but effectively to provide insights where the code’s intent might not be immediately clear.
Practice the DRY Principle
The DRY (Don’t Repeat Yourself) principle emphasizes avoiding repetitive code. Repetition creates unnecessary work and increases the risk of errors. Instead, refactor repeated logic into reusable components or functions. This not only keeps your code concise but also simplifies updates and debugging.
Handle Errors Gracefully
Anticipating and managing errors is critical for a smooth user experience and robust applications. Implement error-handling mechanisms that provide meaningful feedback without disrupting the system. This makes your code more resilient and easier to debug.
Write Tests to Ensure Reliability
Testing is an essential part of clean coding. Unit tests verify that individual components work as intended, while integration tests ensure that the system functions as a whole. A well-tested codebase inspires confidence in its reliability and reduces the likelihood of bugs slipping into production.
Organize Your Code Modularly
Organize your application into modules or components based on functionality. Modularity enhances readability and makes it easier to locate and update specific parts of the code. This practice is particularly valuable in large projects, where complexity can grow rapidly.
Adopt and Enforce a Style Guide
Using a consistent style guide ensures that your code follows established best practices for readability and structure. Style guides provide rules on formatting, naming, and organization, which can be enforced through tools or linters. Adopting these guidelines helps maintain uniformity across the entire codebase.
Refactor Regularly
Refactoring involves revisiting and improving the structure of your code without changing its functionality. Over time, areas of the codebase may become inefficient or outdated. Regularly refactoring keeps your codebase clean, efficient, and aligned with best practices.
Minimize the Use of Global Variables
Global variables can lead to unintended side effects and make debugging challenging. Encapsulate data within functions or classes to limit its scope and reduce dependencies, creating a more predictable and maintainable codebase.
Write Clear Git Commit Messages
In collaborative projects, clear commit messages are invaluable. They should describe what changes were made and why. Avoid generic messages and instead provide context to help others (or yourself) understand the purpose of each change.
Stay Committed to Learning and Adapting
Writing clean code is an ongoing journey. Stay updated on new tools, frameworks, and language features that can enhance your coding practices. Engage with the developer community to exchange ideas and learn from others’ experiences.
Conclusion
Clean code isn’t just about making things work—it’s about making them work beautifully and sustainably. By focusing on clarity, simplicity, and maintainability, you can create code that stands the test of time and collaboration. Adopting these best practices will not only improve your coding skills but also make your work a pleasure for others to read and maintain. Clean code is a shared language of professionalism—embrace it, and your future self will thank you.