A Beginner’s Guide to Functional Programming
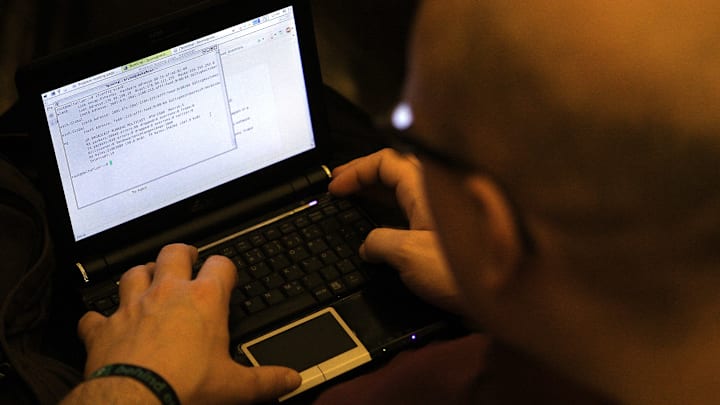
In the sprawling landscape of programming paradigms, functional programming (FP) stands out for its elegant and mathematical approach to problem-solving. Unlike the step-by-step procedural style many programmers start with, FP emphasizes immutability, pure functions, and the seamless composition of smaller functions into larger ones. It’s not just a trend; it’s a transformative way of writing software that prioritizes clarity, reliability, and modularity. Let’s explore how functional programming can reshape the way you think about coding.
What Is Functional Programming?
At its core, functional programming is about building software by composing functions. Unlike object-oriented programming, which revolves around encapsulating data and behavior within objects, FP treats functions as first-class citizens. This means they can be used like any other data type—passed around, stored, or returned by other functions.
However, FP is not just about functions—it’s about pure functions. A pure function produces the same output for the same inputs and doesn’t cause side effects. This means it doesn’t alter external variables, modify databases, or perform other actions that could create unpredictability. This predictability makes FP particularly suited for tasks like parallel processing, debugging, and testing.
Why Functional Programming?
Functional programming isn’t just a theoretical exercise—it provides tangible benefits that can improve the way you build and maintain software.
- Immutability: Data is unchangeable once created. Instead of altering existing structures, FP encourages creating new ones with the necessary changes. Modern programming tools optimize this approach to ensure it’s efficient.
- Ease of Testing: The predictability of pure functions makes them straightforward to test. Without hidden states or dependencies, it’s easier to pinpoint and fix issues.
- Readability and Modularity: Breaking problems into smaller, reusable functions leads to cleaner and more maintainable code.
- Concurrency-Friendly: FP naturally avoids issues like race conditions by eliminating mutable state, making it ideal for concurrent programming.
Key Concepts in Functional Programming
First-Class and Higher-Order Functions
In FP, functions can be passed as inputs to other functions, returned as outputs, or stored in variables. Higher-order functions operate on other functions, either by taking them as arguments or returning them. This flexibility allows you to write concise, reusable code.
Immutability
FP treats data as immutable. Instead of altering a variable or a data structure, FP creates new ones that incorporate the desired changes. This approach eliminates unintended side effects and makes code more predictable.
Pure Functions
Pure functions rely solely on their inputs and produce no side effects. They don't rely on or modify external state, making them easy to test and debug.
Function Composition
One of FP’s greatest strengths is its ability to combine smaller functions into larger ones. Instead of crafting one large, complex function, FP encourages creating a series of smaller, focused functions that work together seamlessly.
Recursion Over Loops
In FP, recursion often replaces traditional looping structures. This approach avoids mutable variables, adhering to the immutability principle while solving problems iteratively.
Languages That Embrace Functional Programming
Some programming languages are designed specifically for functional programming, while others incorporate FP features alongside other paradigms:
- Languages like Haskell and Clojure are examples of purely functional programming languages, built from the ground up to embrace FP principles.
- Languages like Scala and Erlang blend functional and object-oriented programming, offering a middle ground for developers.
- Even popular general-purpose languages like JavaScript and Python include functional programming features, allowing you to adopt FP principles without switching languages entirely.
Getting Started with Functional Programming
If you’re new to FP, begin by applying its principles in a language you’re already familiar with. Start with concepts like writing pure functions, avoiding mutable state, and experimenting with functional utilities provided by your language of choice. Over time, you can explore libraries or frameworks that enhance the functional programming experience.
For a deeper dive, you might explore languages designed for FP, where you can fully immerse yourself in the paradigm and gain a deeper understanding of its advantages.
Embracing the Functional Mindset
Functional programming is more than just a style of coding—it’s a mindset that prioritizes clarity, modularity, and predictability. By breaking problems into smaller, reusable parts and avoiding mutable state, FP encourages a disciplined approach to software development.
Though it may feel unfamiliar at first, the rewards are worth the effort. Functional programming leads to cleaner code, fewer bugs, and a deeper understanding of how your software works. Dive into the world of FP and experience a transformative way to write code. Who knows? It might just become your new favorite paradigm.